Today I lost about four hours debugging what I thought was a bizarre bug due to my own ignorance. Now, I don’t want to be too hard on myself – no one can be an expert in every nook and cranny of a tech stack as large as AppKit. But, still, this one really knocked me on my butt when I realized my mistake.
Tonight I tweeted this…
In today’s exciting episode of Tyler is a Professional Software Developer™…

The bug I was running into happened when I dragged an NSTextField
out of an NSStackView
and dropped it elsewhere in the window. In the gif below you’ll see that after the drop completes, the NSTextField
lingers behind – continuing to duplicate each time I drag and drop it.
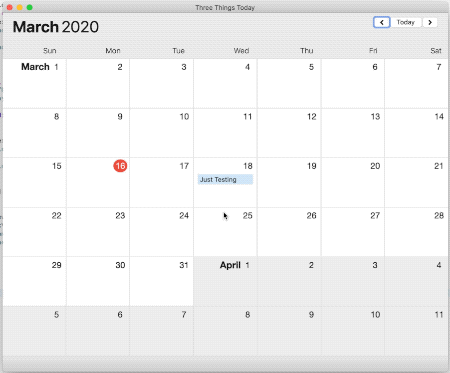
Note: Only the original NSTextField
is draggable. The copies left behind don’t accept mouse events.
So, I start debugging this. My first thought is there’s some sort of race condition happening because when I drop the NSTextField
, the change persists to my Core Data stack – which does the usual NSManagedObjectContext
merge dance and then posts a notification letting the other views in the window know there’s new data and they should refresh. (I don’t know if that’s the proper way to do it, but it’s how I approached it in this situation.)
That notification ? refresh isn’t necessarily anything crazy or complex, but once the change finishes persisting to Core Data, my CloudKit code picks up the new data and pushes it up to the customer’s iCloud account. I don’t just do a push to CloudKit, though. The data model for this app is very, very tiny. So, I’m saving myself some added complexity and just doing an actual two-way sync each time. And, of course, when the sync completes – ? – my views are told to reload any additional changes from the sync session.
I’ve messed up code like this plenty of times before and I’m hoping my first instinct is correct and I’m somehow maybe adding an extra copy of the NSTextField
twice to the NSStackView
?
Here’s the pertinent code. It removes any existing tasks from the NStackView
and then loops through the new data adding a view for each item back into the stack view.
monthDayView.clearTasks()
for t in tasks {
let taskView = t.taskView()
monthDayView.stackView.addArrangedSubview(taskView)
}
Heres’ the implementation for clearTasks()
above:
func clearTasks() {
for view in stackView.arrangedSubviews {
stackView.removeArrangedSubview(view)
}
}
(For the seasoned NSStackView
readers out there who can already see the bug in my code, please hold your laughter while I explain the next frustrating hour of my evening in excruciating detail…)
Seems safe enough, right? But still, my eyes don’t lie. There’s clearly a duplicate NSTextField
hanging around. Let’s dig deeper.
I start with the app in this state:
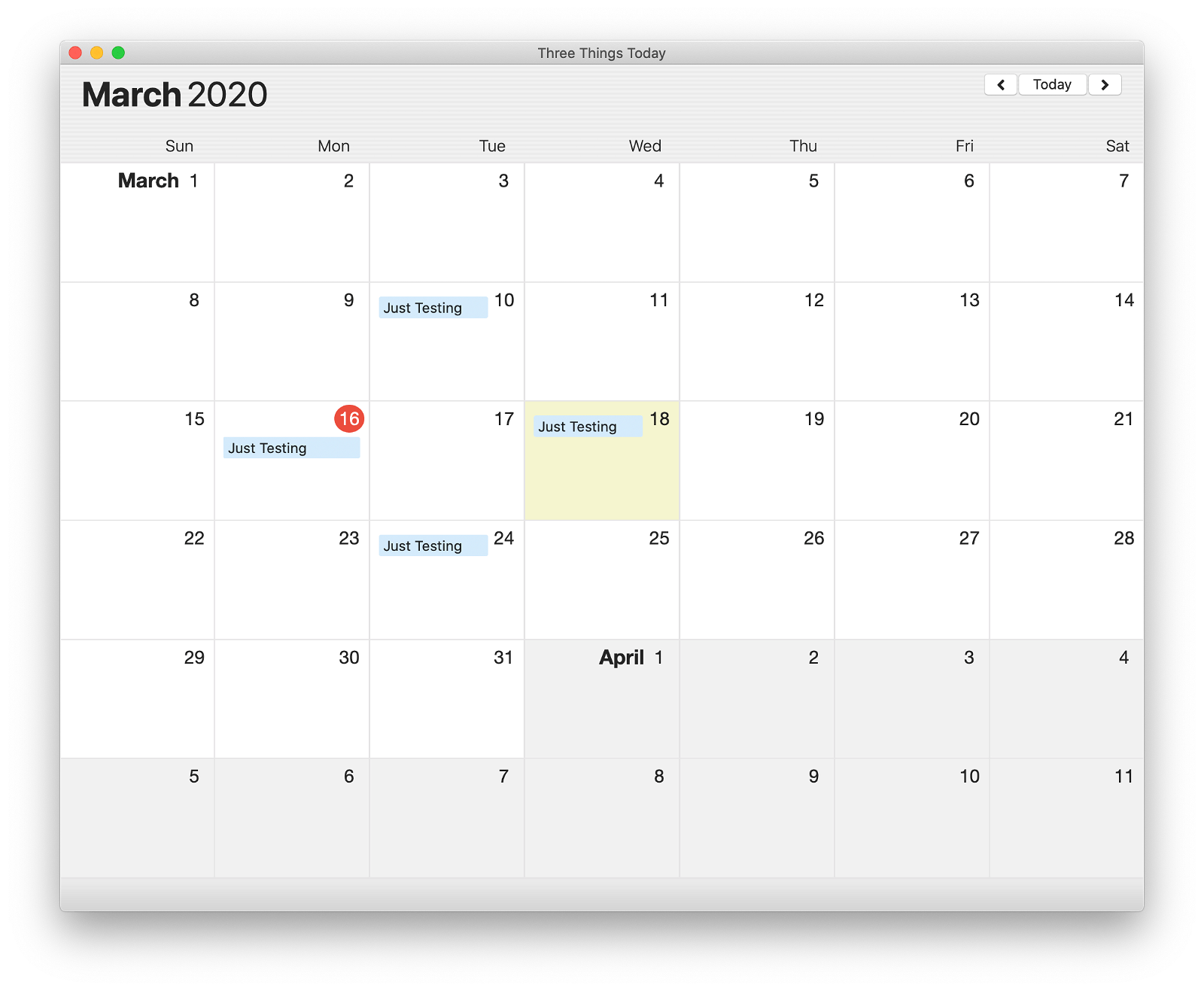
I add this debugging code to confirm if the stack views really do or do not have the number of arranged views I’m expecting:
print(monthDayView.stackView.arrangedSubviews.count)
In the screenshot above, March 18 is the “real” item, and the other three are the weird zombie copies. For each of those views, the above debugging code gives me these results:
- March, 18:
1 views
OK! - March, 10:
0 views
wtf? - March, 16:
0 views
? - March, 24:
0 views
?
Um? That seems…wrong? Those extra views are clearly still there.
Firing up Xcode’s wonderful view debugger, however, completely blew my mind and shattered any remaining self-confidence I had as an app developer…
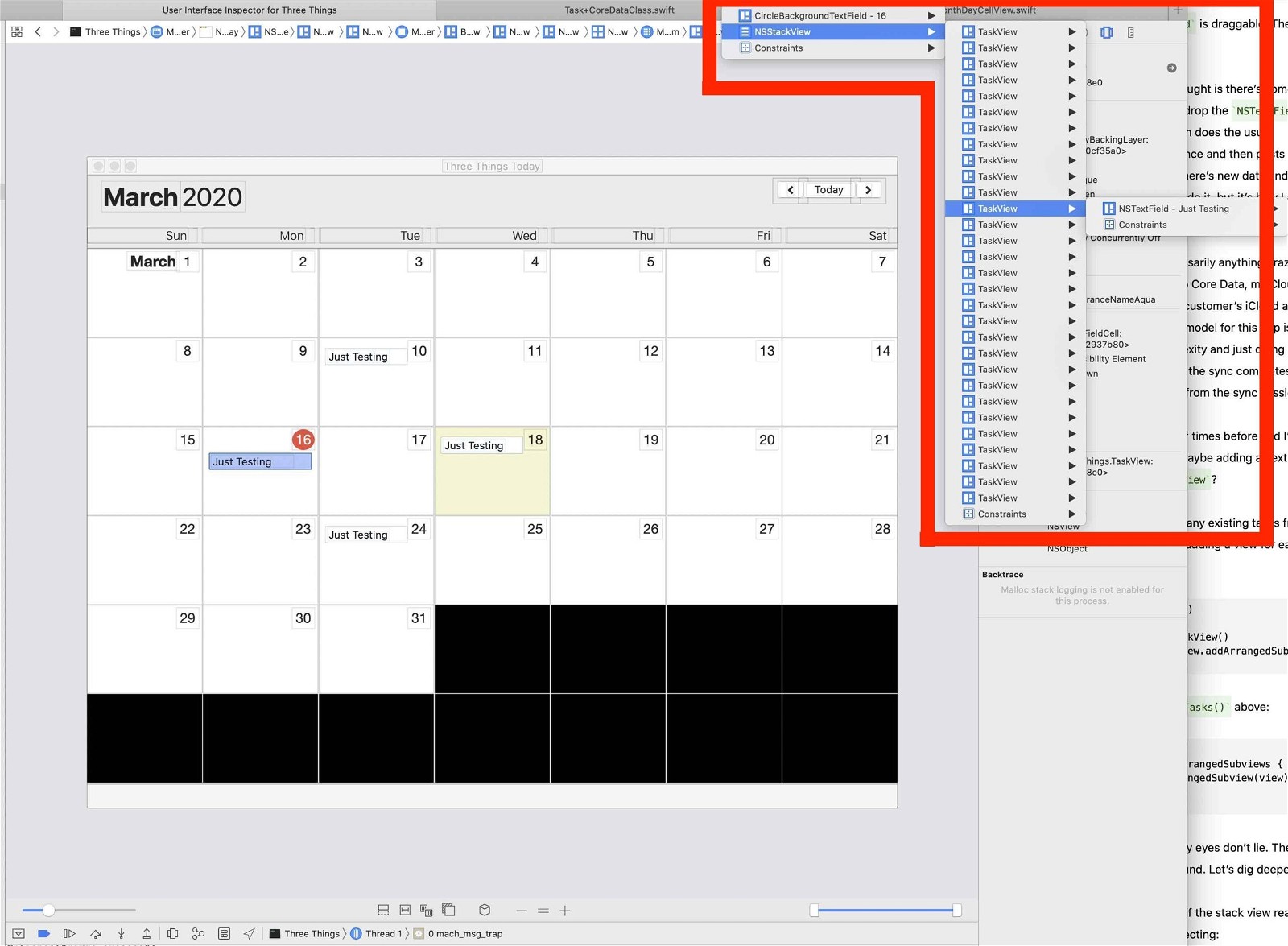
There’s not just one extra NSTextField
hanging about. There. Are. Thirty. Of them.
Clearly at this point I am missing something incredibly obvious and foundational about the situation and frameworks in order for my (I think) relatively simple code to be breaking this badly. Let’s start from first principles and re-read the documentation.
Relatively speaking, NSStackView
is a newish part of AppKit. It’s only been around since Mac OS X (not macOS) 10.9 Mavericks. Regardless, in the seven years since then, I haven’t ever really used it that often. I know it’s there and a nice tool to have available, but I’m just not super familiar with it. And as you’ll soon see, even less so than I thought.
I’m reading through Apple’s documentation in Xcode and I finally stumble upon removeArrangedSubview(_:)
…
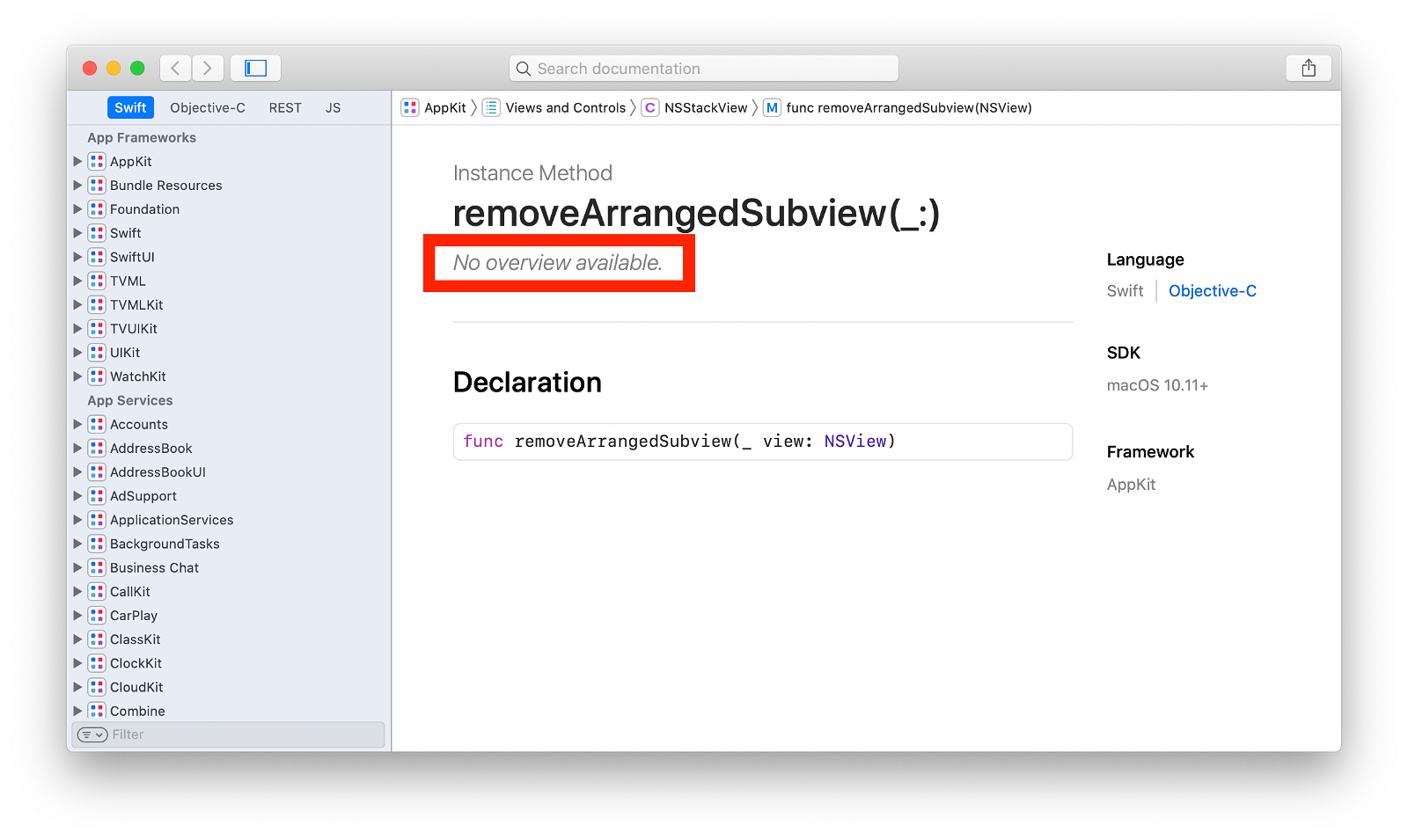
I think that’s strange for a seven year old API, but ok and keep browsing.
Nearly an hour later I’m really questioning everything I thought I knew about ones and zeroes until a google search leads me to this page. And, sure enough, my bug is spelled out right there:
However, using
removeArrangedSubview()
doesn’t remove the view altogether – it keeps the view in memory, which is helpful if you plan to re-add it later on because you can avoid recreating it. Here, though, we actually want to remove the web view and destroy it entirely, and that can be done with a call toremoveFromSuperview()
instead.
Holleeee crap. I never knew stack views worked that way. (Thanks, Paul!) I mean, wow. That is a very basic misunderstanding on my part. So, I add one additional line of code:
func clearTasks() {
for view in stackView.arrangedSubviews {
stackView.removeArrangedSubview(view)
view.removeFromSuperview()
}
}
and ? it works. Not only does it work, but it also fixes a number of other peripheral bugs that I had logged but not investigated yet.
Anyway, I hope this excessively long post has enough keywords stuffed into it so that anyone else facing the same problem can find it.
But, last point. Why didn’t Apple’s documentation mention this very important detail? More so, why isn’t that method documented at all?
Ha, well. Turns out, they did document it. My empty screenshot above is from Xcode’s documentation browser. However, if you go to the same documentation on the developer website you’ll see…
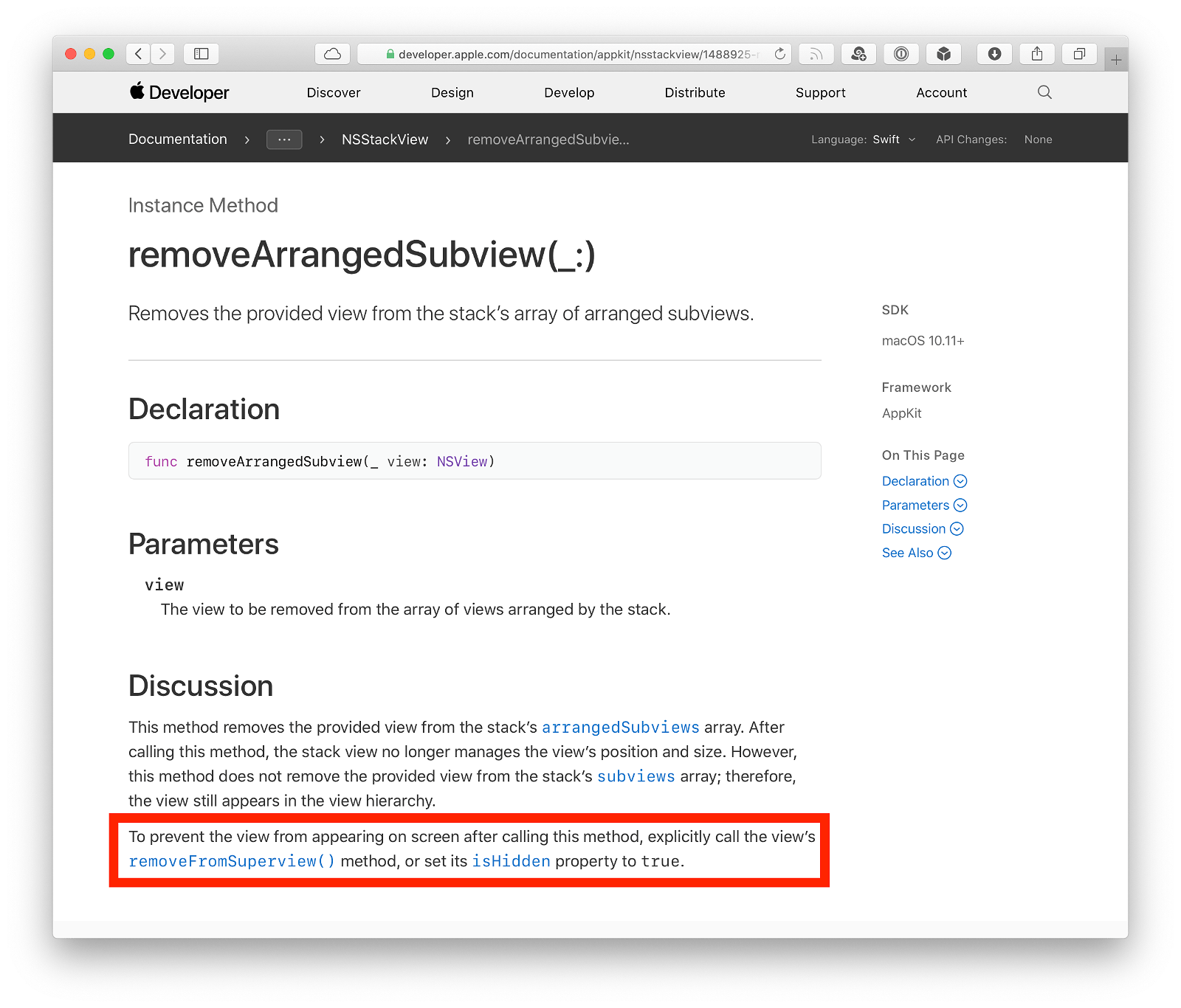
Well played, Apple.